Software concepts- Software concepts encompass a wide range of fundamental principles, techniques, and methodologies used in the development, implementation, and maintenance of software systems. Here are some key software concepts:
- Abstraction: The process of hiding complex implementation details and exposing only necessary functionalities to the user. It helps manage complexity and allows users to work at higher levels of understanding.
- Algorithm: A step-by-step procedure or formula for solving a problem or accomplishing some task. Algorithms are fundamental to computer science and are used in various areas such as sorting, searching, and optimization.
- Data Structures: The organization, storage, and management of data in a computer’s memory or storage. Examples include arrays, linked lists, trees, and graphs.
- Programming Paradigms: Different approaches to programming that dictate the structure, style, and methodology of writing code. Common paradigms include procedural, object-oriented, functional, and declarative programming.
- Design Patterns: Reusable solutions to common problems encountered in software design. Design patterns provide guidelines for designing flexible and maintainable software systems.
- Software Development Life Cycle (SDLC): The process of planning, creating, testing, and deploying software applications. Common SDLC models include Waterfall, Agile, and DevOps.
- Testing: The process of evaluating a software application to ensure that it meets specified requirements and functions correctly. Testing includes unit testing, integration testing, system testing, and acceptance testing.
- Version Control: The management of changes to source code and other files. Version control systems like Git enable multiple developers to collaborate on a project, track changes, and maintain a history of revisions.
- Software Architecture: The structure of a software system, including its components, relationships, and interactions. Architectural patterns such as client-server, microservices, and MVC (Model-View-Controller) help organize and design complex software systems.
- Security: Measures taken to protect software systems from unauthorized access, data breaches, and other security threats. Security concepts include authentication, encryption, access control, and vulnerability management.
- Concurrency: The ability of a system to execute multiple tasks simultaneously. Concurrency concepts involve managing shared resources, synchronization, and avoiding race conditions.
- Performance Optimization: Techniques for improving the speed, efficiency, and resource usage of software applications. Optimization strategies include algorithmic improvements, code refactoring, and profiling.
These concepts form the foundation of software development and are essential for building robust, scalable, and maintainable software systems.
What is Required Software concepts
“Required Software Concepts” typically refers to fundamental concepts and principles that are essential for understanding and working with software in various contexts. These concepts are foundational and necessary for anyone involved in software development, engineering, or related fields. Here are some of the key required software concepts:
- Programming Languages: Understanding programming languages and their syntax, semantics, and paradigms is crucial. This includes concepts like variables, data types, control structures, functions, and classes.
- Data Structures and Algorithms: Knowledge of fundamental data structures (arrays, linked lists, stacks, queues, trees, graphs, etc.) and algorithms (sorting, searching, traversal, dynamic programming, etc.) is essential for efficient problem-solving and software development.
- Software Development Methodologies: Familiarity with various software development methodologies such as Agile, Waterfall, Scrum, and DevOps is necessary for managing projects effectively and delivering high-quality software products.
- Version Control Systems (VCS): Understanding how version control systems like Git work, including concepts such as branching, merging, committing, and resolving conflicts, is essential for collaborative software development and managing codebase history.
- Software Testing: Knowledge of software testing principles, techniques, and methodologies is important for ensuring the quality and reliability of software. This includes unit testing, integration testing, system testing, and test-driven development (TDD).
- Software Design Principles: Understanding software design principles such as SOLID (Single Responsibility, Open/Closed, Liskov Substitution, Interface Segregation, Dependency Inversion), DRY (Don’t Repeat Yourself), KISS (Keep It Simple, Stupid), and YAGNI (You Aren’t Gonna Need It) helps in creating maintainable, scalable, and flexible software architectures.
- Object-Oriented Programming (OOP): Mastery of OOP concepts such as encapsulation, inheritance, polymorphism, and abstraction is essential for building modular, reusable, and extensible software systems.
- Software Architecture Patterns: Knowledge of common software architecture patterns such as client-server, MVC (Model-View-Controller), microservices, and layered architecture helps in designing scalable and maintainable software solutions.
- Concurrency and Parallelism: Understanding concepts related to concurrency (e.g., threads, synchronization, race conditions) and parallelism (e.g., multiprocessing, multithreading, distributed computing) is important for developing efficient and responsive software systems.
- Security Principles: Awareness of security concepts such as authentication, authorization, encryption, input validation, and secure coding practices is essential for protecting software systems against cyber threats and vulnerabilities.
These required software concepts form the basis of a solid understanding of software development and are essential for professionals working in the field of software engineering, development, testing, and related disciplines.
Who is Required Software concepts
My apologies for the misunderstanding. “Required Software Concepts” is not a person; it’s a term used to describe fundamental concepts that are essential for anyone working in the field of software development or engineering. These concepts are necessary knowledge for individuals involved in designing, developing, testing, or maintaining software systems. They form the foundation upon which more advanced skills and knowledge are built.
When is Required Software concepts
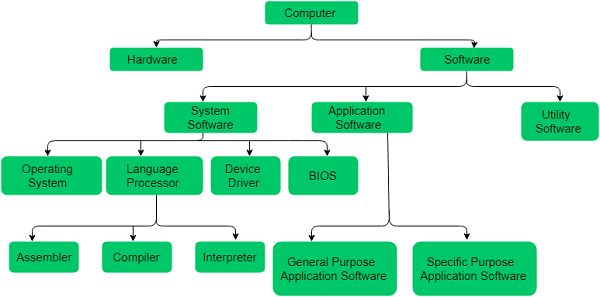
“Required Software Concepts” doesn’t refer to a specific time or date. Instead, it’s a term used to describe fundamental concepts that are necessary for understanding and working with software. These concepts are considered essential regardless of the specific context or time period within the field of software development. They form the basis of knowledge required for individuals to effectively design, develop, test, and maintain software systems. So, “Required Software Concepts” are relevant at all times in the realm of software development.
Where is Required Software concepts
“Required Software Concepts” are not physically located in any particular place; instead, they exist as foundational knowledge within the field of software development. They are typically taught in educational institutions such as universities, colleges, coding bootcamps, and online courses. Additionally, they are acquired through self-study, practical experience, and professional development within the software industry.
These concepts are embedded in textbooks, online resources, documentation, and training materials that are accessible globally. Therefore, they are not confined to any specific location but are rather disseminated and applied wherever software development is practiced.
How is Required Software concepts
“Required Software Concepts” are learned and understood through various means and methods. Here’s how individuals typically acquire and comprehend these fundamental principles:
- Education: Many people learn about software concepts through formal education, such as degree programs in computer science, software engineering, or related fields. Courses in these programs cover foundational concepts like programming languages, data structures, algorithms, and software design principles.
- Self-Study: Individuals often engage in self-directed learning using resources such as books, online tutorials, documentation, and educational websites. Platforms like Coursera, edX, Udacity, and Khan Academy offer courses covering a wide range of software concepts.
- Practice: Hands-on experience is crucial for understanding software concepts. People learn by actually writing code, solving problems, designing software systems, and debugging errors. Personal projects, coding challenges, and open-source contributions provide valuable opportunities for practice.
- Mentorship and Collaboration: Learning from experienced professionals through mentorship, code reviews, pair programming, and collaborative projects can accelerate understanding of software concepts. Interacting with peers in online communities, forums, and meetups also fosters learning and knowledge sharing.
- Work Experience: Working in software development roles exposes individuals to real-world challenges and scenarios, helping them apply and refine their understanding of software concepts. On-the-job learning, training programs, and exposure to diverse projects contribute to professional growth.
- Continuous Learning: The field of software development is constantly evolving, so staying updated on new technologies, tools, and best practices is essential. Engaging in lifelong learning through conferences, workshops, webinars, and online courses ensures that individuals remain proficient in required software concepts.
Overall, mastering “Required Software Concepts” involves a combination of theoretical understanding, practical application, and ongoing learning and adaptation to keep pace with advancements in the field.
Case Study on Software concepts
Development of a Task Management Application
Background: A startup company is developing a task management application to help teams organize their work and improve productivity. The application will allow users to create tasks, assign them to team members, set deadlines, track progress, and communicate within the platform.
Software Concepts Applied:
- Requirements Analysis: Before starting development, the team conducts thorough requirements analysis to understand the needs of the users and stakeholders. They gather requirements through interviews, surveys, and feedback sessions to ensure the application meets user expectations.
- Software Design: Using object-oriented design principles, the team designs the software architecture. They identify key components such as task objects, user authentication modules, database schemas, and communication interfaces. Design patterns like MVC (Model-View-Controller) are employed to separate concerns and improve maintainability.
- Programming Languages and Frameworks: Based on project requirements and team expertise, the developers choose suitable programming languages and frameworks. For example, they might use Python for backend development with Django or Flask framework, and JavaScript with React or Angular for the frontend.
- Data Structures and Algorithms: The team implements data structures such as queues, trees, and graphs to manage tasks efficiently. Algorithms for sorting, searching, and scheduling are applied to optimize task organization and retrieval.
- Version Control: Git is used for version control to manage source code changes. The team follows Git best practices, creating branches for new features or bug fixes, and merging changes through pull requests. This ensures collaboration among team members and maintains a history of code revisions.
- Testing: The application undergoes rigorous testing at various stages of development. Unit tests verify the functionality of individual components, while integration tests ensure that different modules work together seamlessly. Automated testing frameworks like pytest or Jasmine are utilized to streamline the testing process.
- Security: Security measures are implemented to protect user data and prevent unauthorized access. Techniques such as encryption, HTTPS protocol, input validation, and role-based access control (RBAC) are employed to mitigate security risks and ensure compliance with data protection regulations.
- User Experience (UX) Design: The user interface is designed with a focus on usability and intuitiveness. UX principles such as simplicity, consistency, and feedback are applied to enhance user satisfaction and adoption. User testing and feedback loops help refine the interface based on user preferences and behavior.
- Agile Development Methodology: The team adopts Agile methodology to manage project scope and prioritize features. They work in iterative sprints, delivering incremental updates and gathering feedback from stakeholders. Agile ceremonies like daily stand-ups, sprint planning, and retrospective meetings facilitate communication and collaboration within the team.
- Deployment and Continuous Integration/Continuous Deployment (CI/CD): The application is deployed to production environments using CI/CD pipelines. Continuous integration tools like Jenkins or GitLab CI automate the build, test, and deployment process, ensuring that updates are deployed smoothly and reliably.
Outcome: By applying these software concepts effectively, the development team successfully delivers the task management application. The application receives positive feedback from users, who appreciate its intuitive interface, robust features, and reliability. The startup company gains traction in the market and sees increased user adoption, leading to business growth and success.
This case study illustrates how various software concepts are applied throughout the development lifecycle to create a successful software product that meets user needs and business objectives.
White paper on Software concepts
Title: Understanding Software Concepts: A Comprehensive Guide
Abstract:
This white paper serves as a comprehensive guide to understanding fundamental software concepts essential for developers, engineers, and enthusiasts in the field. Software concepts form the bedrock of modern computing, facilitating the creation, deployment, and maintenance of software systems. By elucidating these concepts, this paper aims to empower individuals with the knowledge necessary to navigate the intricacies of software development effectively.
- Introduction to Software Concepts:
- Definition and significance of software concepts
- Evolution of software development practices
- Importance of understanding software concepts in the digital age
- Programming Languages and Paradigms:
- Overview of programming languages and their classification
- Introduction to programming paradigms: imperative, declarative, functional, and object-oriented
- Understanding language syntax, semantics, and execution environments
- Data Structures and Algorithms:
- Introduction to data structures: arrays, linked lists, stacks, queues, trees, graphs, etc.
- Overview of fundamental algorithms: sorting, searching, traversal, dynamic programming, etc.
- Analysis of algorithmic complexity and efficiency
- Software Development Methodologies:
- Comparison of traditional and agile methodologies
- Overview of agile frameworks: Scrum, Kanban, Extreme Programming (XP), etc.
- Best practices in software development lifecycle management
- Version Control and Collaboration:
- Introduction to version control systems (VCS) and their importance
- Overview of Git and other popular VCS platforms
- Collaboration strategies and best practices for distributed development teams
- Software Testing and Quality Assurance:
- Importance of software testing in ensuring product quality
- Overview of software testing types: unit testing, integration testing, system testing, etc.
- Implementation of test-driven development (TDD) and continuous integration (CI)
- Software Design Principles and Patterns:
- Introduction to design principles: SOLID, DRY, KISS, YAGNI, etc.
- Overview of design patterns: creational, structural, and behavioral patterns
- Application of design principles and patterns in real-world scenarios
- Software Architecture and Scalability:
- Understanding software architecture: monolithic vs. microservices, client-server, etc.
- Principles of scalability and performance optimization
- Case studies of scalable software architectures in modern applications
- Security and Privacy:
- Importance of security in software development
- Overview of security concepts: authentication, authorization, encryption, etc.
- Best practices for securing software applications and protecting user data
- Emerging Trends and Technologies:
- Exploration of emerging technologies: artificial intelligence, blockchain, Internet of Things (IoT), etc.
- Implications of emerging trends on software development practices
- Strategies for staying updated and adapting to technological advancements
- Conclusion:
- Recap of key software concepts covered in the paper
- Call to action for continuous learning and professional development
- Future outlook on the evolving landscape of software development
This white paper serves as a comprehensive resource for individuals seeking to deepen their understanding of software concepts and enhance their proficiency in software development practices. By mastering these concepts, stakeholders can navigate the complexities of modern software engineering with confidence and competence.
Industrial Application of Software concepts
Industrial applications of software concepts are pervasive across various sectors, enabling businesses to streamline operations, enhance productivity, and deliver innovative products and services. Here are several examples showcasing how software concepts are applied in different industries:
- Manufacturing and Supply Chain Management:
- Implementation of enterprise resource planning (ERP) systems for inventory management, production scheduling, and resource allocation.
- Utilization of computer-aided design (CAD) and computer-aided manufacturing (CAM) software for product design, prototyping, and manufacturing process optimization.
- Integration of supply chain management software for demand forecasting, procurement, logistics, and distribution.
- Automotive Industry:
- Development of embedded software for vehicle control systems, including engine management, anti-lock braking systems (ABS), and advanced driver-assistance systems (ADAS).
- Integration of software-defined networking (SDN) and Internet of Things (IoT) technologies for vehicle connectivity, remote diagnostics, and over-the-air (OTA) software updates.
- Utilization of computer vision and machine learning algorithms for autonomous driving, object detection, and traffic management.
- Healthcare and Medical Devices:
- Deployment of electronic health record (EHR) systems for patient data management, medical billing, and clinical decision support.
- Development of medical imaging software for diagnostic imaging, image processing, and medical image analysis.
- Integration of wearable devices and health monitoring applications for remote patient monitoring, telemedicine, and personalized healthcare.
- Finance and Banking:
- Implementation of banking software for core banking operations, including account management, transaction processing, and loan origination.
- Utilization of financial analytics software for risk management, portfolio optimization, and algorithmic trading.
- Development of mobile banking applications for digital payments, peer-to-peer transfers, and financial planning.
- Retail and E-commerce:
- Deployment of e-commerce platforms for online storefronts, product catalog management, and customer relationship management (CRM).
- Integration of point-of-sale (POS) software for inventory tracking, sales reporting, and customer loyalty programs.
- Utilization of recommendation engines and personalization algorithms for targeted marketing, product recommendations, and dynamic pricing.
- Energy and Utilities:
- Implementation of supervisory control and data acquisition (SCADA) systems for real-time monitoring and control of energy infrastructure.
- Development of smart grid solutions for energy management, demand response, and renewable energy integration.
- Integration of predictive maintenance software for asset monitoring, condition-based maintenance, and outage management.
- Aerospace and Defense:
- Utilization of simulation software for aircraft design, flight testing, and mission planning.
- Development of embedded software for avionics systems, including flight control, navigation, and communication.
- Integration of cybersecurity solutions for protecting critical infrastructure, securing data transmissions, and mitigating cyber threats.
In summary, software concepts are instrumental in driving innovation and efficiency across diverse industrial sectors, ranging from manufacturing and healthcare to finance and aerospace. By leveraging software technologies and best practices, businesses can gain a competitive edge, improve operational performance, and meet the evolving needs of customers and stakeholders.